Will AI with tools like ChatGPT replace software developers?
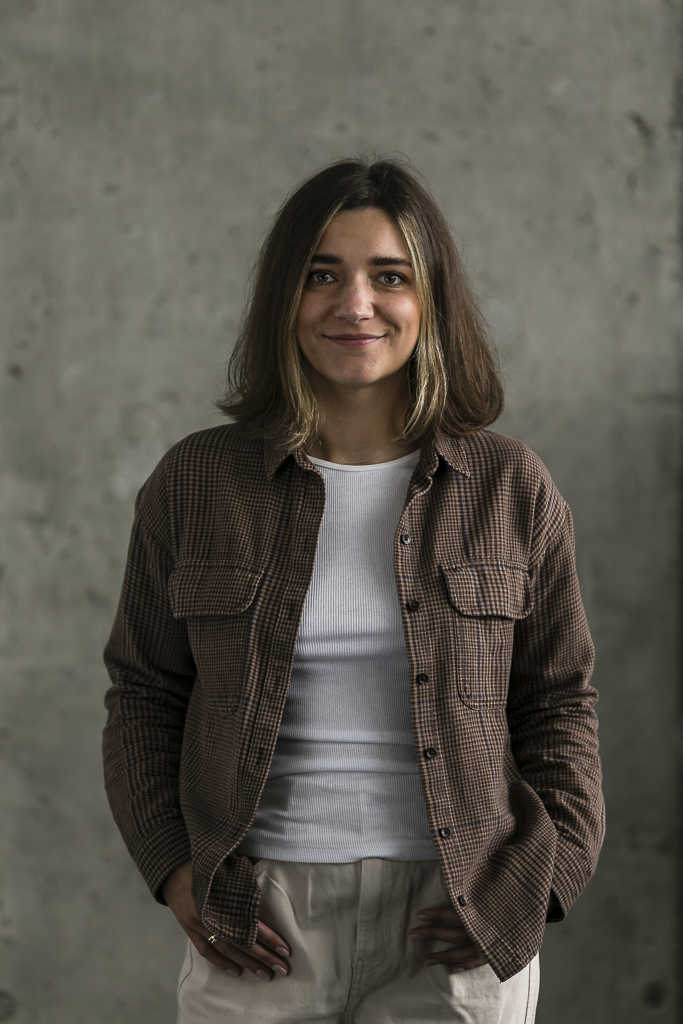
Introduction AI can now produce meaningful lines of code. How cool is that? But should developers be worried about becoming unemployed? Are the creators of AI-powered tools effectively software-designing themselves out of a job? And the main question is what will happen with programmers: should they be afraid of being replaced. Especially if we are […]
The evolution of Material Design. From Material Design to Material You.
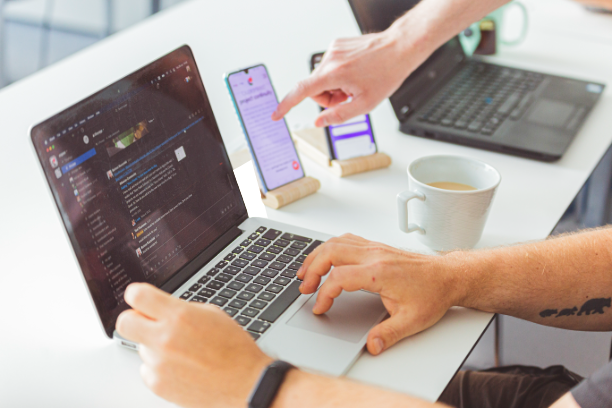
Material Design Evolution
A guide to dependency injection in NestJS
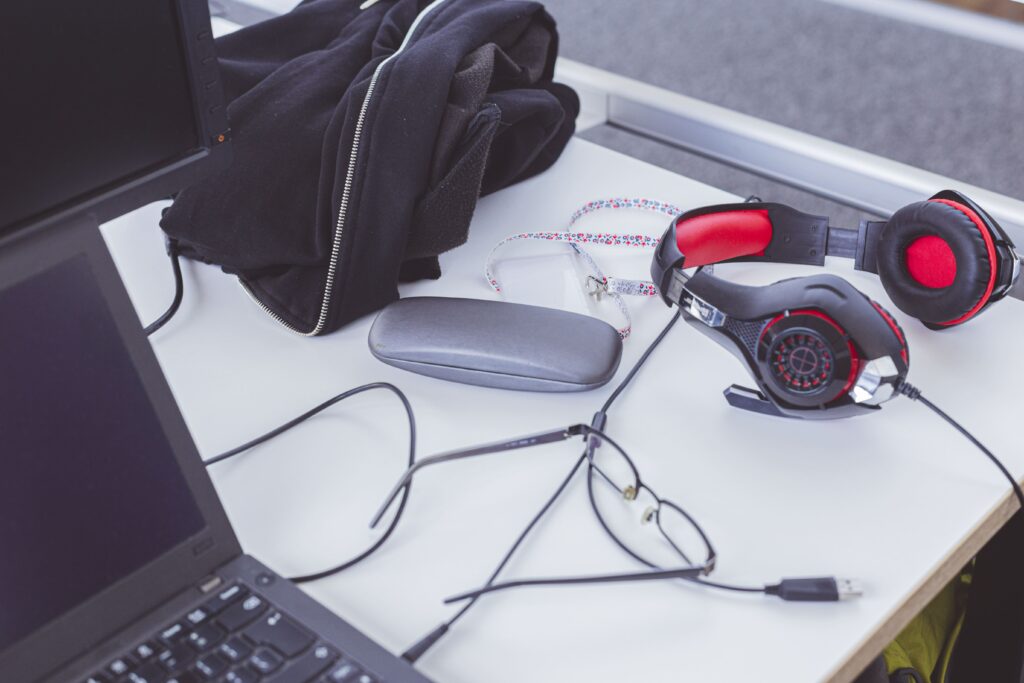
Abstract Dependency injection is one of the most popular patterns in software design. Particularly among developers who adhere to clean code principles. Yet, surprisingly, the differences and relations between the dependency inversion principle, inversion of control, and dependency injection are usually not clearly defined. Therefore, it results in a murky understanding of the subject. The […]
Micronaut vs SpringBoot: which one is better?
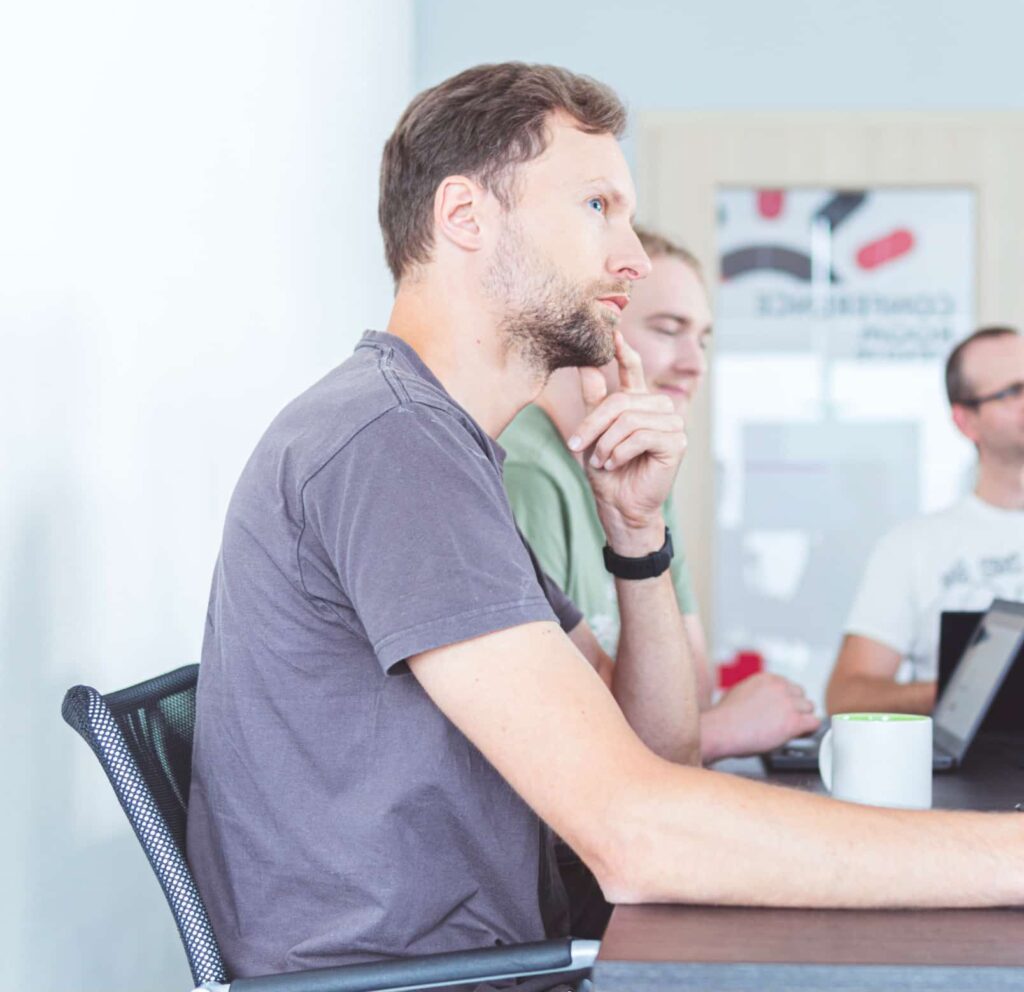
Some time ago, I attended the Devoxx Conference in Kraków, the biggest Java conference in Poland. I searched for inspiration and wanted to hear about the newest trends in the industry. Among many great lectures, one topic left me with a feeling that I should give it more attention – the Micronaut framework and GraalVM […]