An NFT marketplace is an online platform based on blockchain that allows for selling and buying non-fungible tokens (NFT-s). This article will show you how to build and deploy the NFT marketplace from scratch on Polygon. Matic Polygon enables the building of the same applications, consistent with the same standards as Ethereum standards, with the added benefits of lower gas costs and faster transaction speed, among other things.
In the following chapters, we will show you how to build a full-stack application using technologies such as React.js as a front-end framework, Hardhat as a Solidity development environment, IPFS for File Storage, and Web3-React for Polygon Web Client. We have described them briefly below.
Polygon – Polygon is a decentralized Ethereum scaling platform designated to be used for building scalable, user-friendly dApps with low transaction fees. It is compliant with multiple Ethereum security and token standards. The platform offers ready-to-use tools to scale, secure, or build networks.
Metamask – MetaMask is a mature, popular and reliable software cryptocurrency wallet that interacts with the Ethereum blockchain. It allows users to manage their crypto assets through a browser extension or mobile app, which can then be used to interact with decentralized applications. The wallet enables adding multiple EVM network tokens.
React – JavaScript library for rapid development of reactive frontend applications. Mature solution with a growing list of frameworks to build web apps.
Hardhat – Ethereum development environment for creating, testing, and debugging code in Solidity. Flexible and popular in multiple mature project solutions for developers.
IPFS – It is a protocol and peer-to-peer network for distributed storing and sharing files. One of the main IPFS features is content-addressing to uniquely identify each file in a global namespace that connects all devices in the network.
Marketplace smart contract
The central part of the application is the marketplace smart contract “NFTMarketplace” with data storage, core functions, and query functions.
Core functions:
function createMarketItem(address nftContract,uint256 tokenId,uint256 price) payable
function deleteMarketItem(uint256 itemId) public
function createMarketSale(address nftContract,uint256 id) public payable
Query functions:
function fetchActiveItems() public view returns (MarketItem[] memory)
function fetchMyPurchasedItems() public view returns (MarketItem[] memory)
function fetchMyCreatedItems() public view returns (MarketItem[] memory)
A seller can use the smart contract to:
- approve an NFT to market contract
- create a market item with a listing fee
- waiting for a buyer to buy the NFT
- receive the price value
When a buyer buys an NFT in the marketplace, the market contract processes the purchase process:
- buyer buys by paying the price value
- market contract completes the purchase process:
- transfer the price value to the seller
- transfer the NFT from seller to buyer
- transfer the listing fee to the market owner
- change market item state from Created to Release
NFT marketplace smart contract coding
Let’s elaborate on each step with code samples.
Storing data in IPFS
But first of all, let’s create our NFT and store its data on IPFS. IPFS is a peer-to-peer hypermedia protocol designed to preserve and grow humanity’s knowledge by making the web upgradeable, resilient, and more open.
To upload our file to IPFS, let’s use two functions for the front-end side.
The first is uploading an image to the store, and the second uploads metadata with a newly created link to this image.
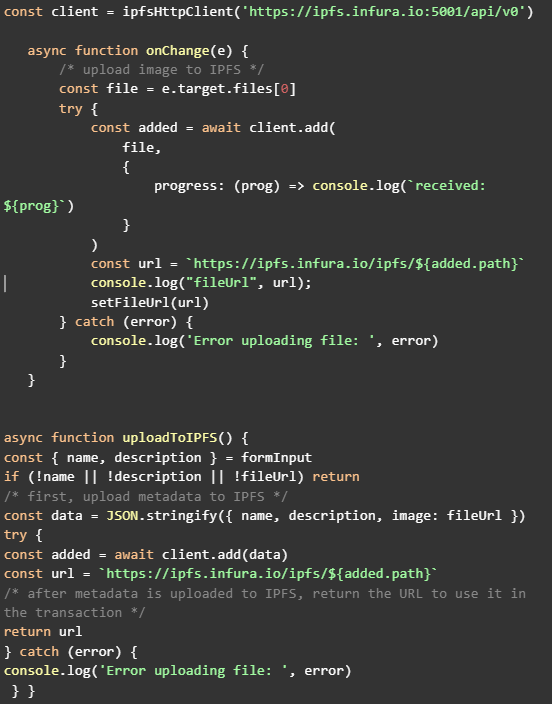
UploadToIPFS function returns url which then we set into NFT ERC721 smart contract.
Minting NFT
We have to create an NFT ERC721 smart contract inheriting OpenZeppelin’s ERC721 implementation. We add such functionality here:
- auto-increment token id
- safeMint(to, tokenId) everyone can mint
- _setTokenURI(tokenId, uri) to set up IPFS URI for our NFT metadata object
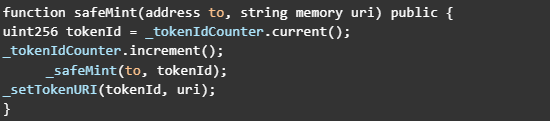
We also add a deploy script scripts/deploy.ts to deploy the smartcontract with name: NFToken and symbol: ERC721

As well as automatically verify the contract script:

As a result, we have just created NFT ERC721 smart contract, deployed it, verified and we can use it to mint multiple NFTs with metadata stored on IPFS.
As blockchain functions, require a time- and resource-consuming mining process of verifying and validating blockchain transactions some time should be gone before we can use our NFT. ERC721 token under the hood emits events we can subscribe for. Let’s subscribe to the Transfer event on our application’s front-end part to get know exactly when/if the transfer or creation of NFT is completed:

And approve this NFT right here to use it by NFTMarketplace smart contract:

NFT marketplace smart contract’s structure
We define a structure for MarketItem:
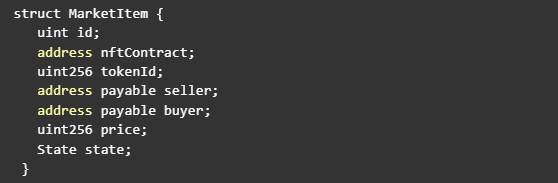
Every market item can be one of three states:

All items are stored in a mapping:

The market has an owner which is the contract deployer. The listing fee will be going to the market owner when an NFT item is sold in the market.
The first thing to do is to list market items for sale by calling createMarketItem function providing a fixed price for it:
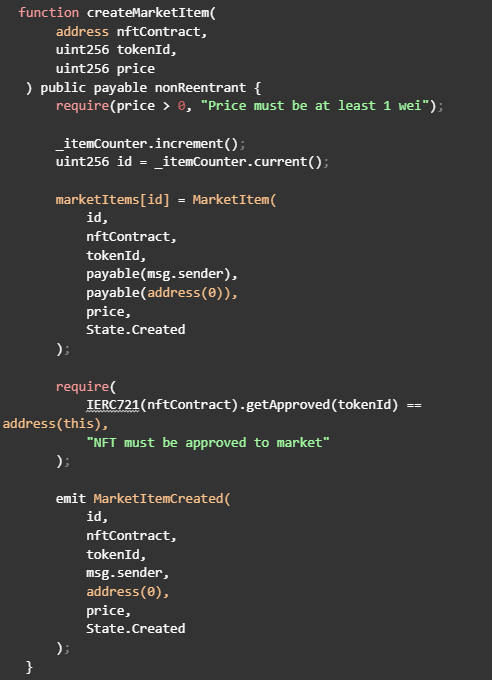
Create MarketItem function also checks whether the price is greater than zero and NFT is approved by the owner of the market. In the end, it emits a creation event to the front-end where we could catch this event for different purposes.
The responsible function for buying is createMarketSale which apart from selling logic checks whether buying price is equal selling price and emits a selling event to the front-end.
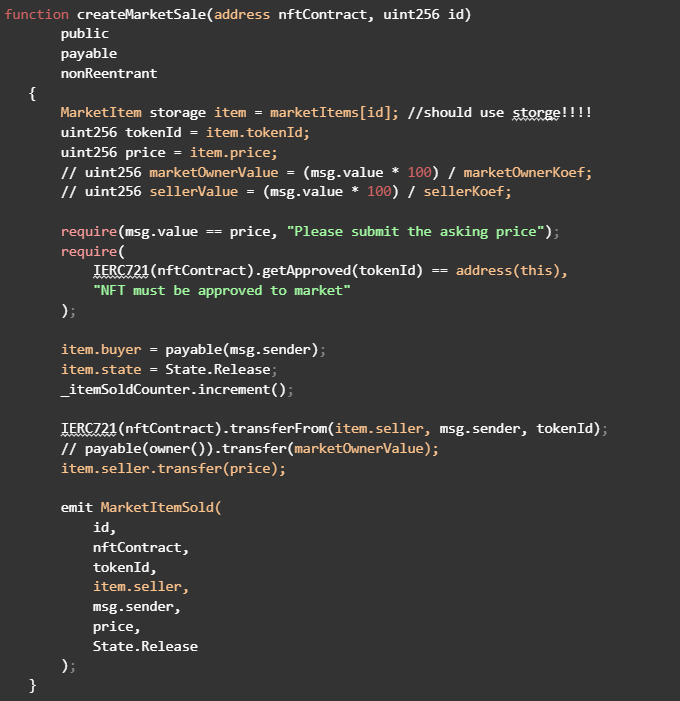
An important part of the application is setting up a market owner fee as well as transferring marketplace ownership:
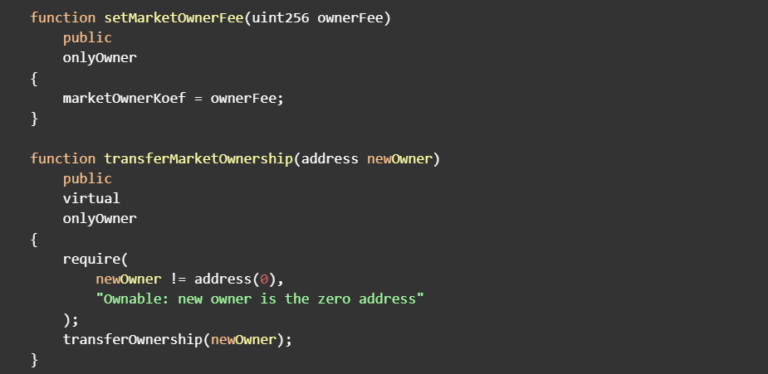
Web3 app setup
Set up web app project using Web3-React & Chakra UI
- React
- Next.js
- Chakra UI
- Web3-React
- ethers.js
- SWR
Crypto wallet configuration
Prepare your MetaMask
Make sure your MetaMask has the RPC URL https://rpc-mumbai.matic.today/ and chain id 80001 for Mumbai Polygon testnet.
In webapp/ folder, run the command from the terminal:

We will use the web3 connecting framework Web3-React to get our job done. The web app stack is:
On the browser, go to page: http://localhost:3000/ where our webpage is running in the local environment and try its functionality.
Summary – Marketplace ready to operate
As a result, we have created a starting point for a customizable alternative to ready-to-use solutions like OpenSea. Notice that we have created not only smartcontract predestined for buying and selling that is crucial for the marketplace. We also made NFTs, so if a collection of NFTs is all you need, use only the smartcontract to mint and get those NFT-s into your wallet.
If there was anything that surprised you as the reader in the process we have described, please feel free to get in touch with us.
This article was written by Blockchain Developer Roman Foltyn. Click HERE for a source code