Micronaut vs SpringBoot: which one is better?
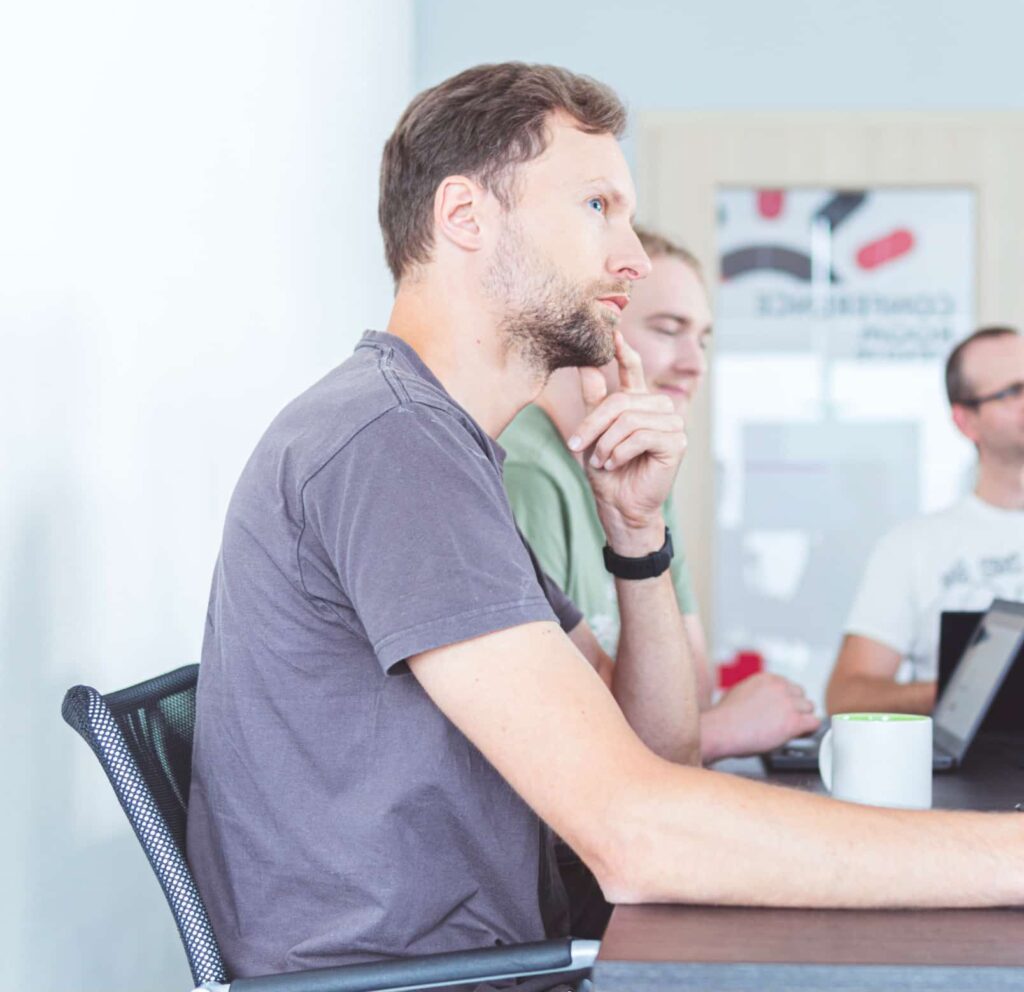
Some time ago, I attended the Devoxx Conference in Kraków, the biggest Java conference in Poland. I searched for inspiration and wanted to hear about the newest trends in the industry. Among many great lectures, one topic left me with a feeling that I should give it more attention – the Micronaut framework and GraalVM […]
Automation testing trends to look out for in 2022
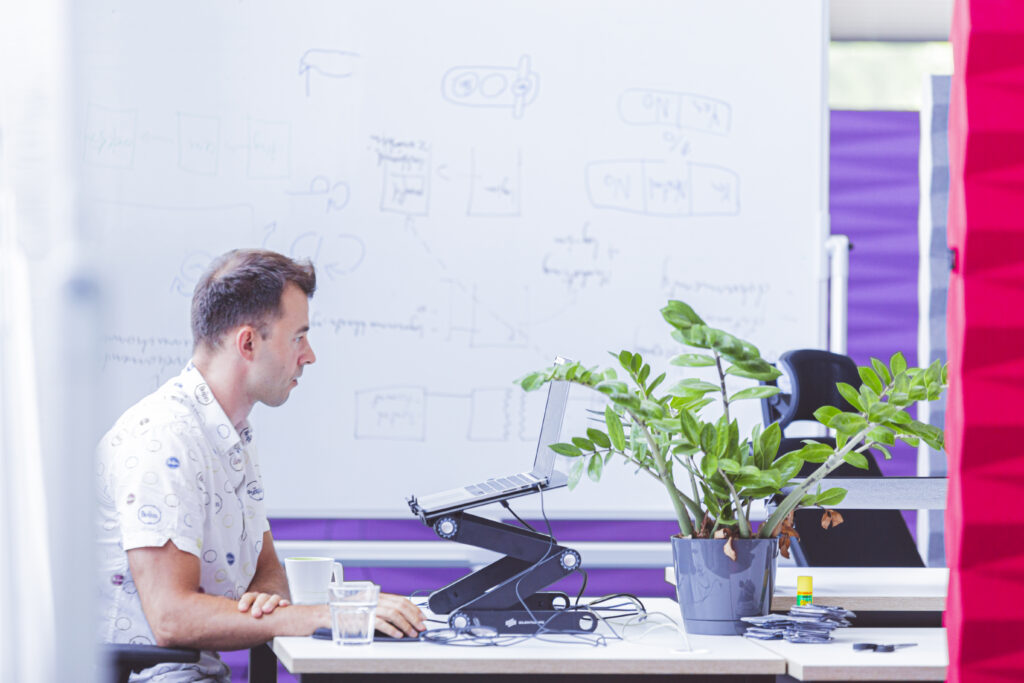
In recent years, the interest in automation testing has grown significantly and despite the fact that manual testing still has a leading position, automation is about to replace it quite soon. Despite the fact that automation testing is an integral part of the software development process, it is becoming more sophisticated in techniques and more […]
The role, skills, and business impact of software architects on product success
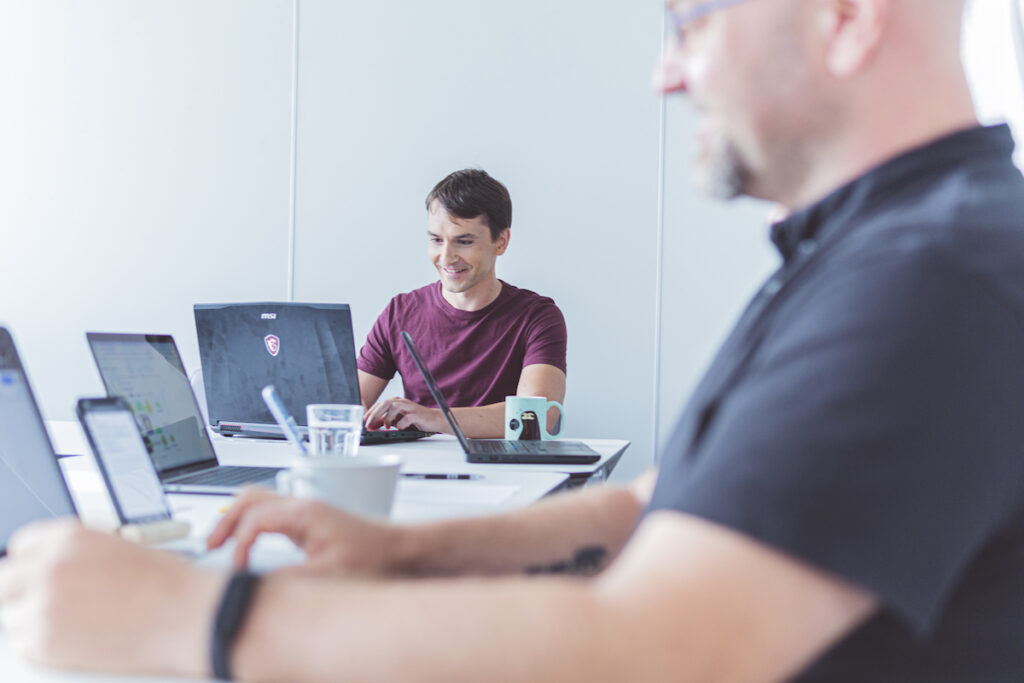
Having well-designed software architecture means having architectural integrity, short-term and strategic guidelines, manageable complexity, and reduced maintenance costs, resulting in an increased business competitiveness. Consequently, well-made decisions on databases, user interfaces, cloud services, and data security guarantee optimal usability of digital solutions. At Espeo Software, we place special emphasis on the architecture in order to […]
How to deploy a Hyperledger Fabric network on Kubernetes?
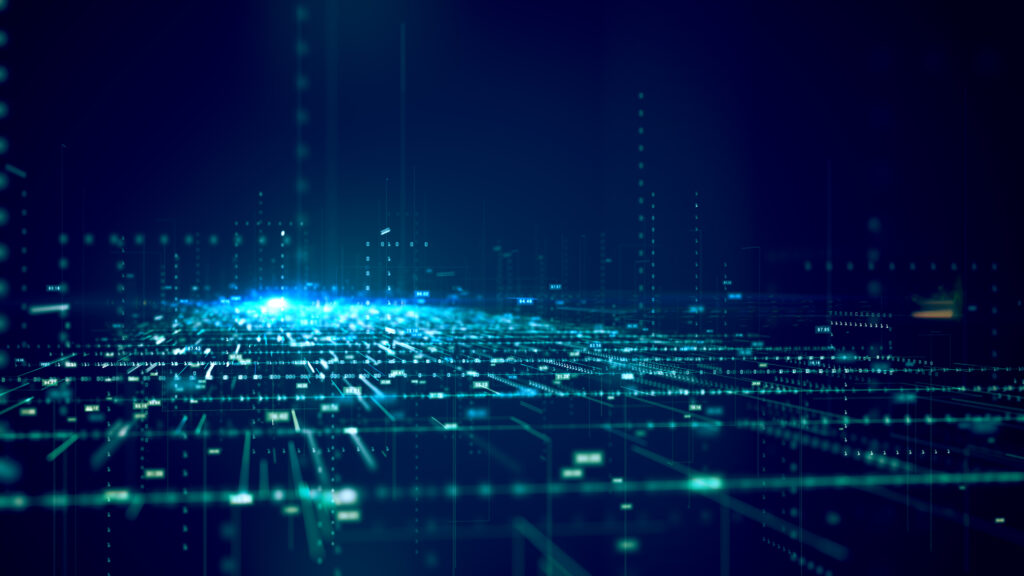
Enterprise-class companies need secure and efficient solutions that can fluently scale with the needs of such large organizations, regardless of the technology of choice. That can be a huge challenge that forces developers to demonstrate their skills, but also the ability to think outside the box and make unobvious decisions. Watch our webinar related to […]